Table Of Content
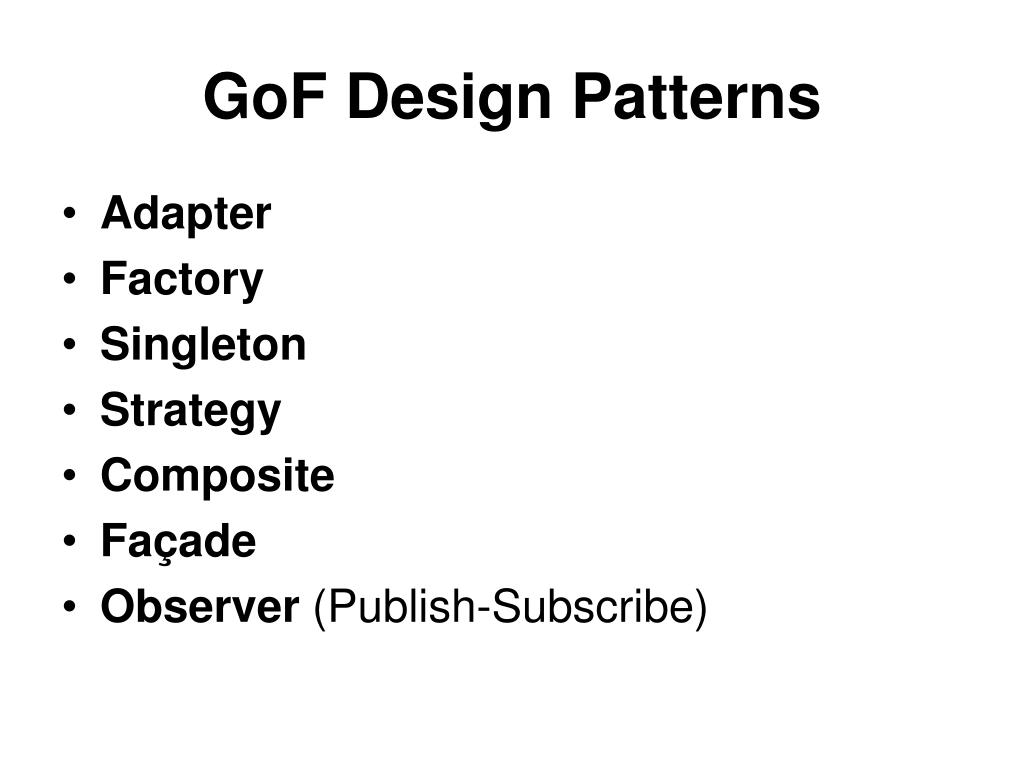
In this example, the Context class holds the strategy to use and can change the strategy at runtime. The AdditionStrategy and SubtractionStrategy classes are concrete strategies that implement different algorithms. The executeStrategy method uses the strategy to calculate the result.
Development and publication history
This was a long one so congratulations if you made it this far. It is very likely that you don’t have to apply all of the patterns but just a few of them, nonetheless it is reasonable to know their existence. Besides, you can always come back to this post to refresh your memory when needed. Turns a request into a stand-alone object that contains all information about the request. This allows for deferred or scheduled execution of operations, queuing of operations, and undo/redo.
Building Better with Creational Patterns
The CareTaker class is used to store and retrieve Memento objects. The basic idea is to allow an object to save its state without exposing the details of its implementation. Enables an application to maintain a large number of objects efficiently.
Iterator
Authorization, feature flipping, rate limiting, and all without changing the commands that handle the requests and in any order you'd like. As the HTTPMiddleware implementors have complete control over if the request continues or not, you can add functionality (almost like decorators) and decide if the request flow continues or not. Proxies are an interface to some other object that might be expensive to create or interact with directly. A typical case for using proxies is in ORM tools when loading an association. You don't necessarily need to load the posts for the user every time you're loading a user so that ORM tools will hide the Posts behind a proxy object.
III. Behavioral patterns
An object can have many types, and objects of different classes can have the same type. Like the Decorator example above, the lack of inheritance also forces proxies to only be available for interface or function types. Design patterns are a fundamental aspect of software design and development. They are reusable solutions to common problems that arise in software design and provide a way for developers to write more maintainable, flexible, and scalable code.
To use this pattern, you would create an instance of the Originator class, set its state, and then create a Memento from the Originator using the saveStateToMemento method. You can then use the CareTaker to store the Memento, and later retrieve it using the get method. To restore the state of the Originator, you would pass the Memento to the getStateFromMemento method. The authors also discuss so-called parameterized types, which are also known as generics (Ada, Eiffel, Java, C#, VB.NET, and Delphi) or templates (C++). These allow any type to be defined without specifying all the other types it uses—the unspecified types are supplied as 'parameters' at the point of use.
Creational Design Patterns
Provides an object that acts as an intermediary for accessing another object. A proxy is used to control access to an underlying object, by providing a surrogate or placeholder for the real object, for example, to add security or caching. When I see patterns in my programs, I consider it a sign of trouble. The shape of a program should reflect only the problem it needs to solve. Consider the distinction between object aggregation and acquaintance and how differently they manifest themselves at compile- and run-times. Aggregation implies that one object owns or is responsible for another object.
Behavioral Patterns
There are 7 design patterns in the Structural Design Patterns category. There are 5 design patterns in the Creational Design Patterns category. In this example, the Context class holds the current state and can change its behavior by changing the state. The ConcreteStateA and ConcreteStateB classes are two concrete states that handle requests differently. The CurrentConditionsDisplay class implements the Observer interface and is used to display the current conditions.
You can write a problem statement and create classes and operations. Or you can model the real world and translate objects found during analysis into design. Design Pattern — Elements of Reusable Object-Oriented Software book describe design patterns using a consistent format. Each pattern is divided into section according to the following template. While you could build strategies in Go using single-method interfaces, that's not very idiomatic. You're better off defining a function signature and having each algorithm being a function that implements the same signature.
Any object can be replaced at run-time by another as long as it has the same type. We say that a type is a subtype of another if its interface contains the interface of its supertype. Gangs of Four design patterns lay the foundation of core design patterns in programming. There are many other design patterns built on top of these patterns for specific requirements. Groups of the fundamental design patterns used in programming are built on four patterns. On top of these design patterns, numerous others are constructed for various requirements.
Decorator pattern dynamically adds/overrides behaviour in an existing method of an object. The functionality of an object is modified at runtime.We can see this pattern being a fundamental piece on frameworks such as Angular or NestJS. The next example shows how to add extra logging when creating a new class. Ensure a class has only one instance, and provide a global point of access to it. A good usage for this pattern is for example when creating a database connection; we want to ensure that connection is not created multiple times but the same instance reused multiple times.
Lets you traverse elements of a collection without exposing the underlying representation of the collection. The pattern defines an iterator interface that includes methods for accessing and manipulating elements of the collection. In this example, we have a Shape interface and a Circle implementation of the Shape interface. The ShapeFactory maintains a pool of Circle objects and reuses them whenever a Circle object of a certain color is requested.
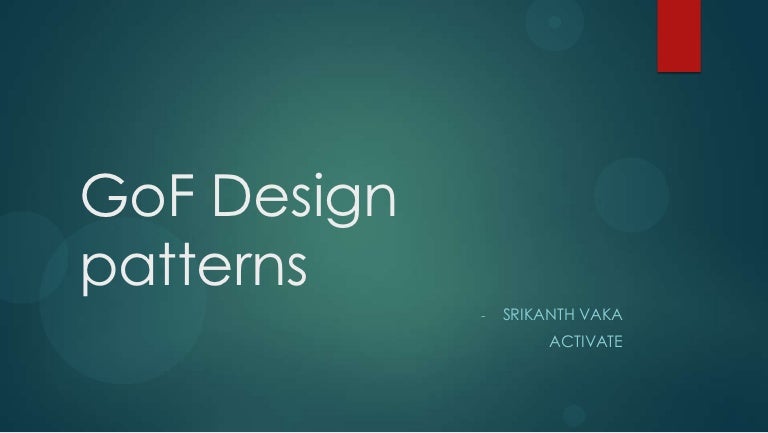
The strategy pattern describes how to implement interchangeable families of algorithms. The state pattern represent each state of an entity as an object. Object oriented design methodologies favor many different approaches.
So much so there have been multiple other books on design patterns, documenting even more examples. You can quickly explain that your solution is an Adapter for anyone who has read about it without detailing what an Adapter is. This pattern separates the construction of a complex object from its representation. The most common motivation for using Builder is to simplify client code that creates these complex objects.
In other words, it provides an interface for creating families of related or dependent objects without specifying their concrete classes. Inheritance and composition each have their advantages and disadvantages. It also make it easier to modify the implementation being reused.
New classes can be defined in terms of existing classes using class inheritance. When a subclass inherits from a parent class, it includes the definitions of all the data and operations that the parent class defines. The second criterion, called scope, specifies whether the pattern applies primarily to classes or to objects. Class patterns deal with relationship between classes and their subclasses. Object patterns deal with object relationship, which can be changed at run-time and are more dynamic. There are 11 behavioral design patterns defined in the GoF design patterns.
No comments:
Post a Comment